Middleware and .NET Core
Middleware, in the context of web development, refers to a software component or a piece of code that sits in the request-response pipeline of a web application. It intercepts and processes HTTP requests and responses. Middleware allows developers to add specific functionalities or behavior to the application in a modular and reusable way. Each middleware component can handle a specific aspect of the request-response flow, such as logging, authentication, caching, CORS, and more.
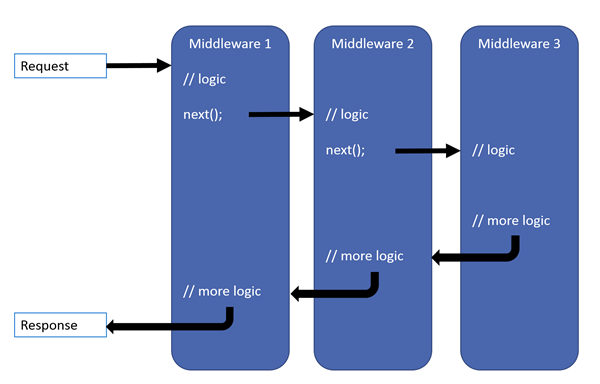
In the context of .NET Core, middleware is a core concept that is integral to the ASP.NET Core framework. It provides inbuilt support for middlewares through the IApplicationBuilder
interface, which is part of the ASP.NET Core application startup process.
When you create an ASP.NET Core web application, the Configure
method in the Startup.cs
file sets up the middleware pipeline. The IApplicationBuilder
interface is passed to this method, and it is used to add various middleware components.
The middleware components are added to the pipeline using extension methods provided by IApplicationBuilder
. These extension methods are found in the Microsoft.AspNetCore.Builder
namespace. Some commonly used built-in middlewares include:
UseRouting
: Sets up routing for the application.UseAuthentication
: Enables authentication middleware.UseAuthorization
: Enables authorization middleware.UseStaticFiles
: Enables serving static files like CSS, JavaScript, and images.UseEndpoints
: Configures the endpoint routing for the application.
For example, in the Configure
method of Startup.cs
, you might have something like this:
public void Configure(IApplicationBuilder app)
{
// Add middleware components to the pipeline
app.UseRouting();
app.UseAuthentication();
app.UseAuthorization();
app.UseStaticFiles();
// ... Other middlewares ...
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
The order in which you add the middleware components matters because each middleware processes the request and can choose to pass it to the next middleware in the pipeline or short-circuit the request and send a response. For example, the UseAuthentication
middleware should come before UseAuthorization
to ensure proper authentication before authorization is performed.
Importance of Middleware Order
In ASP.NET Core, the request processing pipeline for MVC and Razor Pages apps follows a specific order of middleware execution. This order ensures that the request flows through various middleware components, allowing them to process the request and response appropriately. The complete middleware order can be described as follows:
- HTTP Request: The process begins when an HTTP request is received by the ASP.NET Core web server.
- Server Features Middleware: This is the first layer of middleware that runs before anything else. It sets up the server features required for the application, such as handling sockets, upgrading connections, and other low-level server configurations.
- HTTP Logging Middleware: Optionally, you can add logging middleware at this stage to log information about the incoming request.
- HTTP Authentication Middleware: This middleware handles authentication for the application. It checks for authentication schemes and processes authentication-related information like cookies, tokens, or any other custom authentication mechanism.
- Routing Middleware: The routing middleware matches the incoming request’s URL to the appropriate controller and action for MVC or the corresponding Razor Page handler for Razor Pages. It sets up the route data and endpoint information for the request.
- Endpoint Routing Middleware: Starting from ASP.NET Core 3.0, the endpoint routing middleware is used instead of the routing middleware. It provides better performance and a more flexible way to handle routing and endpoint selection.
- Endpoint Middleware: This middleware is responsible for executing the selected endpoint (controller action or Razor Page handler) associated with the matched route.
- Authorization Middleware: After the endpoint is selected, this middleware is executed to check if the user is authorized to access the requested resource based on the specified authorization policies.
- Model Binding Middleware: If the request includes data in the form of query strings, form data, or JSON payloads, this middleware binds that data to the appropriate parameters of the controller action or Razor Page handler.
- Resource Filters and Action Filters: Resource filters and action filters are executed before and after the action execution. They allow you to perform additional processing or validation around the execution of an action method.
- Action Execution Middleware: This middleware executes the selected action method for the request.
- Result Filters: Result filters are executed before and after the execution of the action result. They allow you to modify the result of an action method before it is returned to the client.
- Result Execution Middleware: This middleware handles the execution of the action result, which generates the HTTP response to be sent back to the client.
- Exception Filters: Exception filters are executed if an unhandled exception occurs during the processing of the request. They allow you to handle and log exceptions gracefully.
- HTTP Response Caching Middleware: This middleware caches the HTTP response if caching is enabled for the current request.
- Response Compression Middleware: Optionally, if response compression is enabled, this middleware compresses the HTTP response before sending it back to the client.
- HTTP Response Writing Middleware: This middleware writes the HTTP response back to the client.
- HTTP Response Completion Middleware: This is the last middleware that runs after the response has been sent to the client. It allows you to perform additional tasks after the response has been fully processed.

Please note that the exact middleware order can be influenced by the specific configuration and additional middleware you may have added to the application. The order presented here is the standard order for typical ASP.NET Core MVC and Razor Pages apps.
With this inbuilt support for middleware, ASP.NET Core offers a flexible and robust way to handle various aspects of the request-response pipeline, making it easier to extend and customize the application’s behavior according to specific requirements.
Middleware in .NET Core vs Traditional .NET Framework
Middleware in .NET Core and traditional .NET Framework serve similar purposes, but they are implemented differently due to the architectural differences between the two frameworks.
- Request Pipeline:
- .NET Core: In .NET Core, the request pipeline is based on a modular and lightweight concept of middleware. Each middleware component is a separate unit that processes the HTTP request and response in a chain-like manner.
- Traditional .NET Framework: In the traditional .NET Framework, the request pipeline is typically managed by the ASP.NET pipeline with modules and handlers. Although it can also be extended, the middleware concept is not as lightweight and modular as in .NET Core.
- Hosting Model:
- .NET Core: .NET Core supports cross-platform development and follows a modular and decoupled design philosophy. It is optimized for cloud-based and containerized environments.
- Traditional .NET Framework: The traditional .NET Framework is Windows-specific and relies on Internet Information Services (IIS) as the primary hosting environment.
- Dependency Injection:
- .NET Core: Dependency injection is a first-class citizen in .NET Core. It is built into the framework, and the dependency injection container is readily available for use.
- Traditional .NET Framework: Dependency injection is available but not as deeply integrated as in .NET Core. External libraries or frameworks like Unity or Ninject are often used for dependency injection.
- Cross-Platform Support:
- .NET Core: It is designed to be cross-platform, running on Windows, macOS, and various Linux distributions, allowing applications to be deployed on different platforms.
- Traditional .NET Framework: It is limited to Windows operating systems and is not natively cross-platform.
- Performance:
- .NET Core: Due to its modular and lightweight design, .NET Core typically offers better performance and lower memory footprint compared to the traditional .NET Framework.
- Traditional .NET Framework: While performant, the traditional .NET Framework is generally considered to be less efficient compared to .NET Core, especially in resource-constrained environments.
- Versioning:
- .NET Core: It follows a more aggressive release and versioning cycle, with frequent updates and improvements.
- Traditional .NET Framework: It follows a slower release cycle, and new features are typically introduced with major releases.
Overall, while both middleware concepts achieve the same goal of handling request and response processing, the implementation and philosophy behind middleware in .NET Core differ significantly from the traditional .NET Framework. The move to .NET Core provides developers with more agility, performance, and cross-platform capabilities.
Custom Middleware in .NET Core 6.0
In .NET Core 6.0, a custom middleware is a component that sits in the request pipeline and processes HTTP requests and responses. It allows you to handle specific functionalities like logging, authentication, authorization, CORS (Cross-Origin Resource Sharing), exception handling, and more.
Middleware components are executed in the order they are added to the pipeline, and each middleware can choose to pass the request to the next middleware in the pipeline or short-circuit the request and return a response immediately.
To add a custom middleware in a .NET Core 6.0 application, you can follow these steps:
- Create a custom middleware class: Create a new class that implements the
IMiddleware
interface or use a class that derives fromMiddleware
orMicrosoft.AspNetCore.Http.IMiddleware
class. TheIMiddleware
interface is preferred as it simplifies the middleware implementation by providing a single methodInvokeAsync
.
using System.Threading.Tasks;
using Microsoft.AspNetCore.Http;
public class CustomMiddleware : IMiddleware
{
public async Task InvokeAsync(HttpContext context, RequestDelegate next)
{
// Your custom middleware logic here
// For example, you can modify the request or response, log information, etc.
// Call the next middleware in the pipeline
await next(context);
}
}
- Register the middleware in
Startup.cs
: In theConfigure
method of yourStartup.cs
file, add the custom middleware to the HTTP request pipeline using theUseMiddleware<T>
extension method. Make sure to add it beforeUseEndpoints
to ensure it is executed for every request.
public void Configure(IApplicationBuilder app)
{
// ... Other middlewares ...
app.UseMiddleware<CustomMiddleware>();
// ... Other middlewares ...
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
- Optionally, you can inject services into the custom middleware constructor if needed:
public class CustomMiddleware : IMiddleware
{
private readonly IMyService _myService;
public CustomMiddleware(IMyService myService)
{
_myService = myService;
}
public async Task InvokeAsync(HttpContext context, RequestDelegate next)
{
// Your custom middleware logic here using _myService
// ...
await next(context);
}
}
By following these steps, you’ve successfully added a custom middleware to your .NET Core 6.0 application. Remember that the middleware order matters and the sequence in which you add them can affect how the requests are processed in the pipeline.